A. Lib using soundmanager2
B. step by step
1. download lib soundmanager2
http://www.schillmania.com/projects/soundmanager2/
2. create project
- ionic start myApp blank --v2
- create folder public add file soundmanager2.js to folder
- Script using
import { Injectable, ViewChild } from '@angular/core';
import { Http } from '@angular/http';
import 'rxjs/add/operator/map';
import { Categorys, Playlists } from '../models/user';
import { Observable } from 'rxjs/Rx';
import { soundManager } from '../public/js/soundmanager2';
@Injectable()
export class MusicPlayerService {
currentTrack;
isPlaying: boolean;
trackProgress = 0;
trackList = [];
currentPosition;
IdSongplay: any;
constructor(public http: Http) {
this.http = http;
this.init();
}
init() {
this.initSoundManager();
}
playTrack(track) {
if (typeof this.getCurrentTrack() !== 'undefined') this.stop();
var _this = this;
this.setCurrentTrack(track);
var foo = soundManager.createSound({
id: track.id,
url: track.src,
multiShot: false
});
foo.options.whileplaying = function () {
this.IdSongplay = this.id;
//console.log("ID:" + this.id);
/**time lap */
var min = (this.durationEstimate / 1000 / 60) << 0,
sec = Math.round((this.durationEstimate / 1000) % 60);
var sect = (sec < 10) ? '0' + sec.toString() : sec.toString();
var mint = (min < 10) ? '0' + min.toString() : min.toString();
var timeplay = "<sm> [" + mint + ':' + sect + "]</sm>";
/* Play Model*/
var titlesong = document.getElementById('nameSong');
if (titlesong != null)
titlesong.innerHTML = track.title + timeplay;
var titlesong1 = document.getElementById('nameSong1');
if (titlesong1 != null)
titlesong1.innerHTML = track.title + timeplay;
var x = document.getElementById('progBar');
if (x != null)
x.setAttribute('style', 'width:' + ((this.position / this.duration) * 100) + '%');
var x1 = document.getElementById('progBarplay');
if (x1 != null)
x1.setAttribute('style', 'width:' + ((this.position / this.duration) * 100) + '%');
/*Play List music */
var playfooter = document.getElementById('playfooter');
if (playfooter != null)
playfooter.setAttribute('style', 'display:block;');
var titlesongf = document.getElementById('nameSongf');
if (titlesongf != null)
titlesongf.innerHTML = track.title + timeplay;
var titlesongf1 = document.getElementById('nameSongf1');
if (titlesongf1 != null)
titlesongf1.innerHTML = track.title + timeplay;
var xf = document.getElementById('progBarf');
if (xf != null)
xf.setAttribute('style', 'width:' + ((this.position / this.duration) * 100) + '%');
var x1f = document.getElementById('progBarf');
if (x1f != null)
x1f.setAttribute('style', 'width:' + ((this.position / this.duration) * 100) + '%');
/*Play list catalog */
var playfooterlc = document.getElementById('playfootercat');
if (playfooterlc != null)
playfooterlc.setAttribute('style', 'display:block;');
var titlesonglc = document.getElementById('nameSonglc');
if (titlesonglc != null)
titlesonglc.innerHTML = track.title + timeplay;
var titlesonglc1 = document.getElementById('nameSonglc1');
if (titlesonglc1 != null)
titlesonglc1.innerHTML = track.title + timeplay;
var xlc = document.getElementById('progBarlc');
if (xlc != null)
xlc.setAttribute('style', 'width:' + ((this.position / this.duration) * 100) + '%');
var x1lc = document.getElementById('progBarlc');
if (x1lc != null)
x1lc.setAttribute('style', 'width:' + ((this.position / this.duration) * 100) + '%');
/*button icon */
/* if (this.isPlaying) {
//play
var xf = document.getElementById('Idconplay');
if (xf != null) {
xf.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xf.setAttribute('ng-reflect-name', 'ios-play-outline');
xf.setAttribute('aria-label', 'ios-play-outline');
}
//List play
var xfp = document.getElementById('Idconplaylist');
if (xfp != null) {
xfp.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xfp.setAttribute('ng-reflect-name', 'ios-play-outline');
xfp.setAttribute('aria-label', 'ios-play-outline');
}
//List cate
var xfc = document.getElementById('Iconplaylistlc');
if (xfc != null) {
xfc.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xfc.setAttribute('ng-reflect-name', 'ios-play-outline');
xfc.setAttribute('aria-label', 'ios-play-outline');
}
}
else {
//play
var xf = document.getElementById('Idconplay');
if (xf != null) {
xf.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xf.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xf.setAttribute('aria-label', 'pause outline');
}
//List play
var xfp = document.getElementById('Idconplaylist');
if (xfp != null) {
xfp.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xfp.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xfp.setAttribute('aria-label', 'pause outline');
}
//List cate
var xfc = document.getElementById('Iconplaylistlc');
if (xfc != null) {
xfc.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xfc.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xfc.setAttribute('aria-label', 'pause outline');
}
}*/
}
foo.options.onfinish = function () {
/* Play Model*/
var x = document.getElementById('progBar');
if (x != null)
x.setAttribute('style', 'width:' + 0 + '%');
/*Play List music */
var x1f = document.getElementById('progBarf');
if (x1f != null)
x1f.setAttribute('style', 'width:' + 0 + '%');
/*Play List catalog */
var x1lc = document.getElementById('progBarlc');
if (x1lc != null)
x1lc.setAttribute('style', 'width:' + 0 + '%');
console.log("onfinish");
_this.nextTrack();
}
foo.play();
this.setPlayingStatus(true);
}
play() {
soundManager.play(this.getCurrentTrack().id);
this.setPlayingStatus(true);
}
pause() {
soundManager.pause(this.getCurrentTrack().id);
this.setPlayingStatus(false);
}
stop() {
this.pause();
this.resetProgress();
soundManager.stopAll();
soundManager.unload(this.getCurrentTrack());
soundManager.destroySound(this.getCurrentTrack().id);
}
resetProgress() {
this.trackProgress = 0;
}
togglePlay() {
if (this.isPlaying) {
this.pause();
/*play*/
var xf = document.getElementById('Idconplay');
if (xf != null) {
xf.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xf.setAttribute('ng-reflect-name', 'ios-play-outline');
xf.setAttribute('aria-label', 'ios-play-outline');
}
/*List play*/
var xfp = document.getElementById('Idconplaylist');
if (xfp != null) {
xfp.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xfp.setAttribute('ng-reflect-name', 'ios-play-outline');
xfp.setAttribute('aria-label', 'ios-play-outline');
}
/*List cate*/
var xfc = document.getElementById('Iconplaylistlc');
if (xfc != null) {
xfc.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xfc.setAttribute('ng-reflect-name', 'ios-play-outline');
xfc.setAttribute('aria-label', 'ios-play-outline');
}
}
else {
this.play();
/*play*/
var xf = document.getElementById('Idconplay');
if (xf != null) {
xf.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xf.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xf.setAttribute('aria-label', 'pause outline');
}
/*List play*/
var xfp = document.getElementById('Idconplaylist');
if (xfp != null) {
xfp.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xfp.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xfp.setAttribute('aria-label', 'pause outline');
}
/*List cate*/
var xfc = document.getElementById('Iconplaylistlc');
if (xfc != null) {
xfc.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xfc.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xfc.setAttribute('aria-label', 'pause outline');
}
}
}
checkPlay() {
console.log("check play:" + this.isPlaying);
if (!this.isPlaying) {
console.log("check play change icon");
/*play*/
var xf = document.getElementById('Idconplay');
if (xf != null) {
xf.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xf.setAttribute('ng-reflect-name', 'ios-play-outline');
xf.setAttribute('aria-label', 'ios-play-outline');
}
/*List play*/
var xfp = document.getElementById('Idconplaylist');
if (xfp != null) {
xfp.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xfp.setAttribute('ng-reflect-name', 'ios-play-outline');
xfp.setAttribute('aria-label', 'ios-play-outline');
}
/*List cate*/
var xfc = document.getElementById('Iconplaylistlc');
if (xfc != null) {
xfc.setAttribute('class', 'icon icon-md ios-play-outline ion-ios-play-outline');
xfc.setAttribute('ng-reflect-name', 'ios-play-outline');
xfc.setAttribute('aria-label', 'ios-play-outline');
}
}
else {
/*play*/
var xf = document.getElementById('Idconplay');
if (xf != null) {
xf.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xf.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xf.setAttribute('aria-label', 'pause outline');
}
/*List play*/
var xfp = document.getElementById('Idconplaylist');
if (xfp != null) {
xfp.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xfp.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xfp.setAttribute('aria-label', 'pause outline');
}
/*List cate*/
var xfc = document.getElementById('Iconplaylistlc');
if (xfc != null) {
xfc.setAttribute('class', 'icon icon-md ion-ios-pause-outline');
xfc.setAttribute('ng-reflect-name', 'ion-ios-pause-outline');
xfc.setAttribute('aria-label', 'pause outline');
}
}
}
setPlayingStatus(playing: boolean) {
this.isPlaying = playing;
}
getPlayingStatus(): boolean {
return this.isPlaying;
}
setCurrentTrack(track) {
this.currentTrack = track;
}
getCurrentTrack() {
return this.currentTrack;
}
getCurrentPosition() {
return this.currentPosition;
}
getdurationEstimate() {
if (typeof this.getCurrentTrack() === 'undefined') return 0;
var sound = soundManager.getSoundById(this.getCurrentTrack().id);
return sound.durationEstimate;
}
setCurrentPosition(position) {
this.currentPosition = position;
}
getDuration(position) {
if (typeof this.getCurrentTrack() === 'undefined') return 0;
var sound = soundManager.getSoundById(this.getCurrentTrack().id);
return sound.durationEstimate;
}
nextTrack() {
if (typeof this.getCurrentTrack() === 'undefined'
|| this.trackList.length < 1)
return;
this.stop();
console.log(this.trackList.indexOf(this.getCurrentTrack()));
var nextPosition = this.trackList.indexOf(this.getCurrentTrack()) + 1;
if (this.trackList.length > nextPosition) {
this.playTrack(this.trackList[nextPosition]);
} else {
this.playTrack(this.trackList[0]);
}
}
prevTrack() {
if (typeof this.getCurrentTrack() === 'undefined'
|| this.trackList.length < 1)
return;
this.stop();
//console.log(this.trackList.indexOf(this.getCurrentTrack()));
var prevPosition = this.trackList.indexOf(this.getCurrentTrack()) - 1;
if (prevPosition > 0) {
this.playTrack(this.trackList[prevPosition]);
} else {
this.playTrack(this.trackList[0]);
}
}
addToPlaylist(track) {
this.trackList.push(track);
}
clearPlaylist() {
this.trackList = [];
}
initSoundManager() {
var _this = this;
soundManager.setup({
preferFlash: false, // prefer 100% HTML5 mode, where both supported
debugMode: false, // enable debugging output ($log.debug() with HTML fallback)
useHTML5Audio: true,
onready: function () {
//$log.debug('sound manager ready!');
},
ontimeout: function () {
alert('SM2 failed to start. Flash missing, blocked or security error?');
alert('The status is ' + this.status.success + ', the error type is ' + this.status.error.type);
},
defaultOptions: {
// set global default volume for all sound objects
autoLoad: true, // enable automatic loading (otherwise .load() will call with .play())
autoPlay: false, // enable playing of file ASAP (much faster if "stream" is true)
from: null, // position to start playback within a sound (msec), see demo
loops: 1, // number of times to play the sound. Related: looping (API demo)
multiShot: false, // let sounds "restart" or "chorus" when played multiple times..
multiShotEvents: true, // allow events (onfinish()) to fire for each shot, if supported.
onid3: null, // callback function for "ID3 data is added/available"
// onload: true, // callback function for "load finished"
onstop: null, // callback for "user stop"
onfailure: function () {
_this.nextTrack();
}, // callback function for when playing fails
onpause: null, // callback for "pause"
onplay: null, // callback for "play" start
onresume: null, // callback for "resume" (pause toggle)
position: null, // offset (milliseconds) to seek to within downloaded sound.
pan: 0, // "pan" settings, left-to-right, -100 to 100
stream: true, // allows playing before entire file has loaded (recommended)
to: null, // position to end playback within a sound (msec), see demo
type: 'audio/mp3', // MIME-like hint for canPlay() tests, eg. 'audio/mp3'
usePolicyFile: false, // enable crossdomain.xml request for remote domains (for ID3/waveform access)
volume: 100, // self-explanatory. 0-100, the latter being the max.
whileloading: function () {
},
whileplaying: function () {
_this.currentPosition = ((this.position / this.duration) * 100);
},
onfinish: function () {
_this.nextTrack();
},
onload: function () {
if (this.readyState == 2) {
console.log('Error loading track');
_this.nextTrack();
}
}
}
});
}
/*Tinhs giay */
Timelable: any;
totalTime(input) {
var min = (input / 1000 / 60) << 0,
sec = Math.round((input / 1000) % 60);
this.Timelable = this.pad(min) + ':' + this.pad(sec);
}
pad(d) {
return (d < 10) ? '0' + d.toString() : d.toString();
}
}
link app: https://play.google.com/store/apps/details?id=com.dproject.radiozing
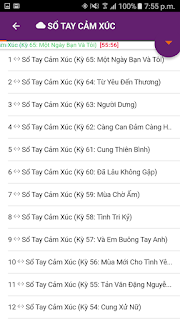
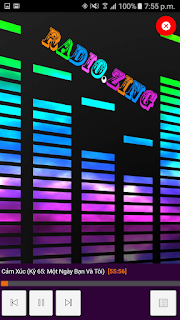
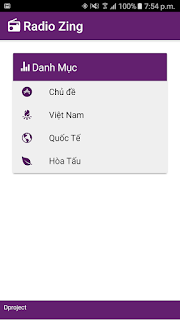
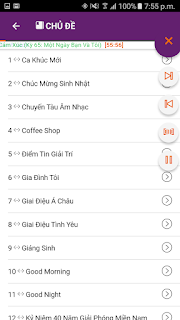
Full source code available?
ReplyDeleteI was busy that today ,I can answer you
Deletelink app full vs.0.0.3: http://yamechanic.com/1NNh
thanks.. (h) (h) (h)
Delete